How to make a custom view in Kotlin
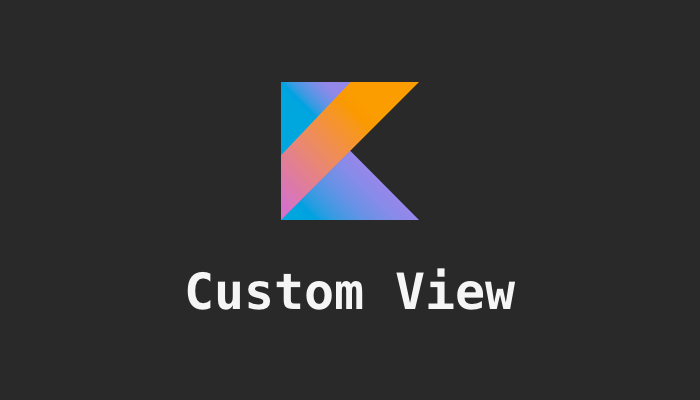
To create a custom view in Kotlin, you can create a new subclass of View
and override the onDraw
method to draw the view's content. Then, you can add any additional properties or behavior that you want the view to have.
Here is an example of a custom view class in Kotlin:
class CustomView(context: Context, attrs: AttributeSet?) : View(context, attrs) {
private val paint = Paint()
init {
// Customize the appearance of the view here
paint.color = Color.BLACK
paint.style = Paint.Style.STROKE
paint.strokeWidth = 2.0f
}
override fun onDraw(canvas: Canvas?) {
// Draw the view's content here
canvas?.drawCircle(100f, 100f, 50f, paint)
}
// Add any additional methods or behavior here
}
In this example, the CustomView
class is a subclass of View
that has a private paint
property. The init
block is used to customize the appearance of the view, and the onDraw
method is overridden to draw the view's content (a circle in this case).
To use the custom view, you can create an instance of the CustomView
class and add it to your layout, just like any other view:
val view = CustomView(context)
view.layoutParams = ViewGroup.LayoutParams(100, 100)
viewGroup.addView(view)
You can also add any additional methods or behavior to the custom view class, such as handling touch events or updating the appearance of the view based on its state.