Popup Custom View in UIKit
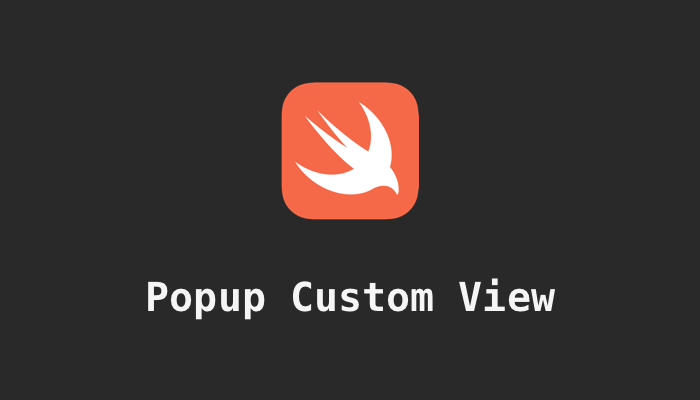
In UIKit, popup mainly can be:
- popup an alert using
UIAlertController
- popup action sheet using
UIAlertController
. For more details, check this: Present action sheet in UIKit and SwiftUI - popup a custom view/viewController
The following will demonstrate how to present a custom view/viewController by creating a custom popup by subclassing a UIViewController
and presenting it modally.
Here is an example of how to create a custom popup in UIKit:
// custom popup viewcontroller
class PopupViewController: UIViewController {
var dismissHandler: (() -> Void)?
override func viewDidLoad() {
super.viewDidLoad()
// Customize the appearance of the view here
view.backgroundColor = .white
}
@IBAction func dismissButtonTapped(_ sender: Any) {
dismissHandler?()
dismiss(animated: true, completion: nil)
}
}
// How to use
class ViewController: UIViewController {
@IBAction func showPopupButtonTapped(_ sender: Any) {
let popup = PopupViewController()
popup.dismissHandler = {
// Update the UI or perform any other actions when the popup is dismissed
}
present(popup, animated: true, completion: nil)
}
}
In this example, the PopupViewController
is a UIViewController
subclass that represents the content of the popup. It has a dismissHandler
property that is used to update the UI or perform any other actions when the popup is dismissed.
The ViewController
class has a showPopupButtonTapped
action method that is called when the button is tapped. It creates an instance of the PopupViewController
and presents it modally.
To dismiss the popup, the dismiss(_:animated:completion:)
method is called on the PopupViewController
. The dismissHandler
closure is executed before the popup is dismissed, and the popup is removed from the view hierarchy.
You can customize the appearance and behavior of the popup by adding additional views or controls to the PopupViewController
, or by using a custom modal presentation style.