Singleton in Kotlin
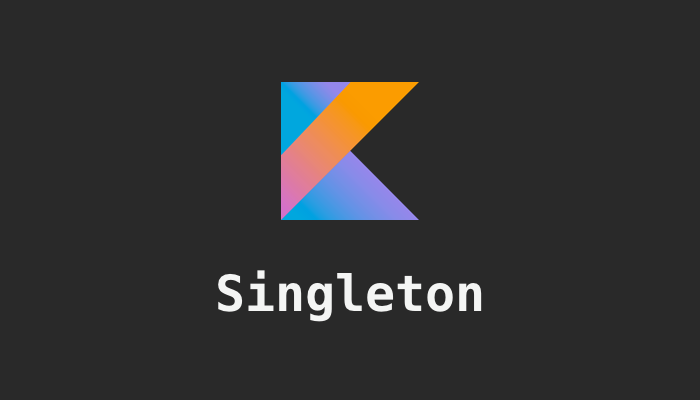
In the Kotlin programming language, a singleton is a design pattern that allows you to create only one instance of a class. This is useful when you want to make sure that there is only one instance of a particular class, for example, if you want to create a global object that can be accessed from anywhere in your code. Here is an example of how you might implement a singleton in Kotlin:
class Singleton private constructor() {
companion object {
private var instance: Singleton? = null
fun getInstance(): Singleton {
if (instance == null) {
instance = Singleton()
}
return instance
}
}
}
In this example, we have a class called Singleton
that has a private constructor, which means that it cannot be instantiated directly. Instead, we have a companion object with a static method called getInstance()
that is used to create and return the single instance of the Singleton
class. The first time getInstance()
is called, it creates a new instance of Singleton
and stores it in a static variable called instance
. Subsequent calls to getInstance()
simply return the instance that was created the first time.