Type safe in programming language
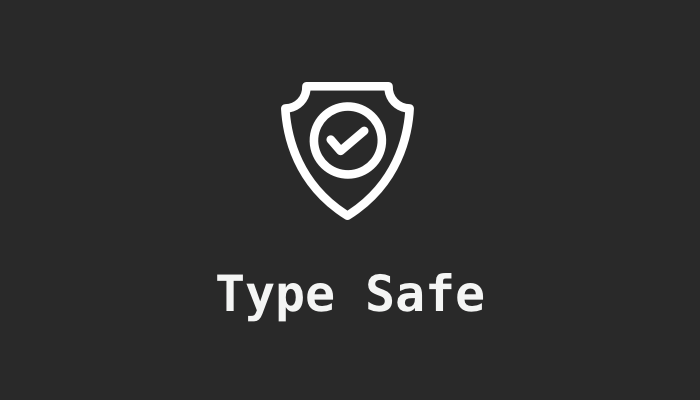
Introduction
Type safety in programming languages refers to the concept that variables and data structures are strongly typed and cannot be assigned values of different types. This means that the programming language will not allow the programmer to accidentally or intentionally assign a incorrect type to a variable or data structure later on in a program, which helps us to prevent errors and ensure the reliability and stability of the program.
Type safe vs. Strongly typed
These two are related concepts. Type safe is as mentioned above, while Strongly typed meaning a variable has a certain type and will not change in a program at any time.
Type safe Example in Swift
Type safety is a key feature of the Swift language, as it can help us to ensure that code is correct and predictable by enforce both variables and constants are checked at compile time.
As many of us heard that "Swift is designed to be type safe", in other words, Swift compiler will not allow us to perform operations on variables that are not compatible with their defined type. For example, if you have a variable of type Int
(an integer value), you cannot perform operations on it that expect a String
(a sequence of characters) value. If you try to do this, the Swift compiler will generate an error and prevent your code from being executed. See the following code:

Unlike other programming languages (such as Python), Type safe is a quite helpful feature in Swift. As it not only helps to prevent many common programming errors but also makes it easier to write code that is predictable and reliable. Think about this, we are enforcing strict rules on the types of values that can be used in different situations in the very beginning, Swift will do the rest jobs to ensure that your code will behave as you expect it to and will avoid unexpected or incorrect results.
Type safe Example in Kotlin
Similarly, Type safety is also a concept in the programming language Kotlin. Like Swift, Kotlin is a type-safe language, which means that all values have a specific type and it is not possible to use values of the wrong type in your code. This helps prevent errors and ensures that your code behaves as expected.
Let us have a look at an example of how type safety works in Kotlin:
val myString = "Hello, world!"
val myInt = 42
// The following line of code would cause an error, because
// you cannot add a string and an integer together.
//val result = myString + myInt
// Instead, you would need to convert the integer to a string
// in order to add it to the other string.
val result = myString + myInt.toString()
In the above example, we have a string variable and an integer. And in the following line, we are trying to add those two values together. Obviously it is not possible as they are not the same type as we all know.