Use keystore to store your sensitive in Kotlin
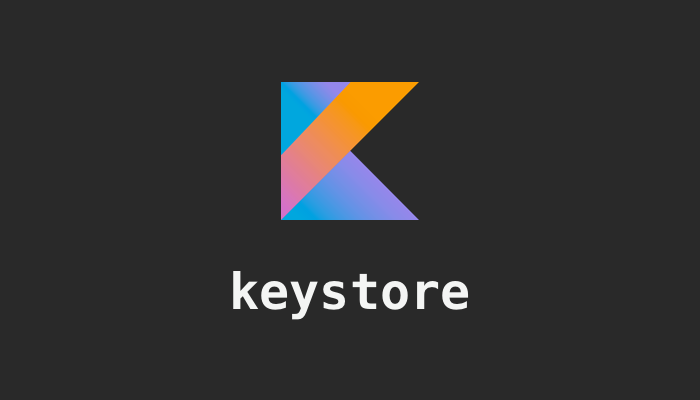
The keystore is a secure storage location in Android that is used to store encryption keys, digital certificates, and other sensitive information. The keystore is encrypted with a hardware-based key, which makes it much more difficult for an attacker to access the stored data.
To use the keystore in Kotlin, you can use the KeyStore
class from the java.security
package. Here is an example of how to use the keystore to store and retrieve a symmetric key:
import java.security.KeyStore
import javax.crypto.Cipher
import javax.crypto.KeyGenerator
val keyStore = KeyStore.getInstance("AndroidKeyStore")
keyStore.load(null)
// Generate a symmetric key
val keyGenerator = KeyGenerator.getInstance("AES", "AndroidKeyStore")
keyGenerator.init(256)
val key = keyGenerator.generateKey()
// Encrypt the data
val cipher = Cipher.getInstance("AES/GCM/NoPadding")
cipher.init(Cipher.ENCRYPT_MODE, key)
val encryptedData = cipher.doFinal("hello".toByteArray())
// Decrypt the data
cipher.init(Cipher.DECRYPT_MODE, key)
val decryptedData = cipher.doFinal(encryptedData)
In this example, we use the KeyGenerator
class to generate a symmetric key, and the Cipher
class to encrypt and decrypt the data. The key is stored in the keystore and is encrypted with the device's hardware-based key.
It is important to note that the keystore is a secure storage location, but it is not a secure communication channel. If you need to transmit sensitive data over a network, you should use a secure protocol like HTTPS.