Certificate pinning and Public key pinning in Kotlin
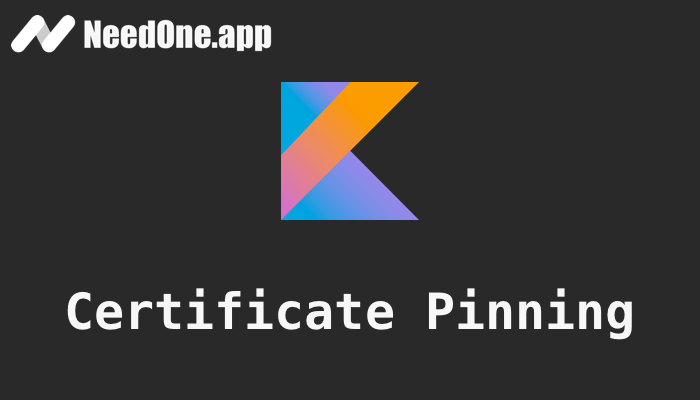
With the last article (Certificate pinning and Public key pinning), we know the concept of Certificate pinning and Public key pinning, and how to implement them in iOS. This article will demonstrate how to implement them in Kotlin.
Implement certificate pinning
Certificate pinning is a security technique that involves associating a specific certificate or public key with a particular server. This helps to prevent man-in-the-middle attacks by ensuring that the client only trusts the specific certificate or public key associated with the server, and not any other certificate that may be presented by an attacker.
Here is an example of how to implement certificate pinning in Kotlin using the OkHttp library:
Implement public key pinning
val certificatePinner = CertificatePinner.Builder()
.add("example.com", "sha256/1234567890ABCDEFGHIJKLMNOPQRSTUVWXYZ")
.build()
val client = OkHttpClient.Builder()
.certificatePinner(certificatePinner)
.build()
val request = Request.Builder()
.url("https://example.com")
.build()
client.newCall(request).execute()
In this example, we create a CertificatePinner
object using the CertificatePinner.Builder
class. We add a pin for the example.com
domain using the add
method, specifying the domain name and the SHA-256 hash of the public key.
Next, we create an OkHttpClient
object using the OkHttpClient.Builder
class and set the CertificatePinner
object using the certificatePinner
method.
Finally, we create a Request
object using the Request.Builder
class and specify the URL of the server. We use the OkHttpClient
object to send the request and execute it.