Lazy in Kotlin
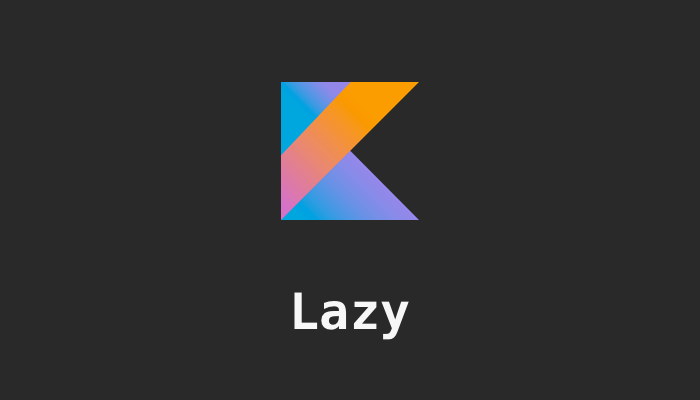
In the Kotlin programming language, the term "lazy" typically refers to a type of property or variable that is only initialized when it is first accessed, rather than when the containing object is created. This can be useful for optimizing the performance of an application, by delaying the creation of costly objects until they are actually needed.
For example, consider the following code that defines a LazyProperty
class with a lazy
property called val
:
class LazyProperty {
val lazy: String by lazy {
"Hello"
}
}
In this case, the lazy
property will only be initialized when it is first accessed, at which point the lazy
block will be executed to compute its value. This means that if the lazy
property is never accessed, the expensive computation will never be performed, which can save resources and improve performance.
You can also specify additional parameters for the by lazy
delegate, such as the mode
which determines whether the property should be initialized eagerly (i.e., when the containing object is created) or lazily (i.e., when the property is first accessed). For example:
class LazyProperty {
val lazy: String by lazy(mode = LazyThreadSafetyMode.SYNCHRONIZED) {
// some expensive computation
"Hello"
}
}
n this case, the lazy
property will be initialized using the SYNCHRONIZED
thread safety mode, which means that multiple threads can safely access the property without the need for explicit synchronization.
Benefits of using Lazy in Kotlin
There are several benefits to using lazy properties in Kotlin, including improved performance and reduced memory usage.
One of the main benefits of lazy properties is that they can improve the performance of an application by delaying the initialization of costly objects until they are actually needed. For example, if you have an object that takes a long time to create or requires a lot of memory, you can use a lazy property to defer its creation until it is first accessed. This can save resources and improve the overall performance of the application.
Another benefit of lazy properties is that they can help to reduce memory usage by only creating objects when they are actually needed. This is because lazy properties are only initialized when they are first accessed, and are then cached for future use. This means that if a lazy property is never accessed, the object it references will never be created, which can save memory.
Additionally, lazy properties can make your code easier to read and understand, by explicitly separating the initialization of an object from its usage. This can make it clear when and how an object is being created, which can help to prevent mistakes and improve the maintainability of your code.
Overall, lazy properties can be a useful tool for optimizing the performance and memory usage of your Kotlin application, and can make your code easier to read and understand.
Drawbacks of using Lazy in Kotlin
While lazy properties can offer many benefits, there are also some potential drawbacks to consider.
One potential drawback of lazy properties is that they can make your code more complex, by requiring you to use the by lazy
delegate and specify a lambda expression for initializing the property. This can add additional overhead to your code, and can make it more difficult to understand for other developers who are not familiar with the concept of lazy properties.
Another potential drawback is that lazy properties can make it more difficult to test your code, since the initialization of a lazy property is deferred until it is first accessed. This can make it challenging to ensure that the property has been properly initialized in your test cases, and can require you to use additional tools or techniques to verify its behavior.
Additionally, lazy properties can introduce potential race conditions in your code if they are not used carefully. For example, if multiple threads try to access a lazy property concurrently, it is possible that the property will be initialized multiple times, which can lead to unexpected behavior and potential errors.
Overall, while lazy properties can offer many benefits, it is important to consider these potential drawbacks and use them carefully in order to avoid potential problems.