An action sheet is a component that displays a set of choices to the user in a modal format.
UIKit
In iOS, you can create an action sheet using a UIAlertController
with the .actionSheet
style.
Here is an example of how to create an action sheet in Swift:
let actionSheet = UIAlertController(title: "Choose an option", message: nil, preferredStyle: .actionSheet)
let option1 = UIAlertAction(title: "Option 1", style: .default) { _ in
// Handle the selection of option 1
}
let option2 = UIAlertAction(title: "Option 2", style: .default) { _ in
// Handle the selection of option 2
}
let cancel = UIAlertAction(title: "Cancel", style: .cancel) { _ in
// Handle the cancellation of the action sheet
}
actionSheet.addAction(option1)
actionSheet.addAction(option2)
actionSheet.addAction(cancel)
present(actionSheet, animated: true, completion: nil)
In this example, the actionSheet
variable is a UIAlertController
with the .actionSheet
style. The UIAlertAction
objects represent the choices that are displayed in the action sheet, and can be customized with different styles (.default
, .cancel
, .destructive
, etc.) and handler blocks to respond to user selections.
This will display the above action sheet.
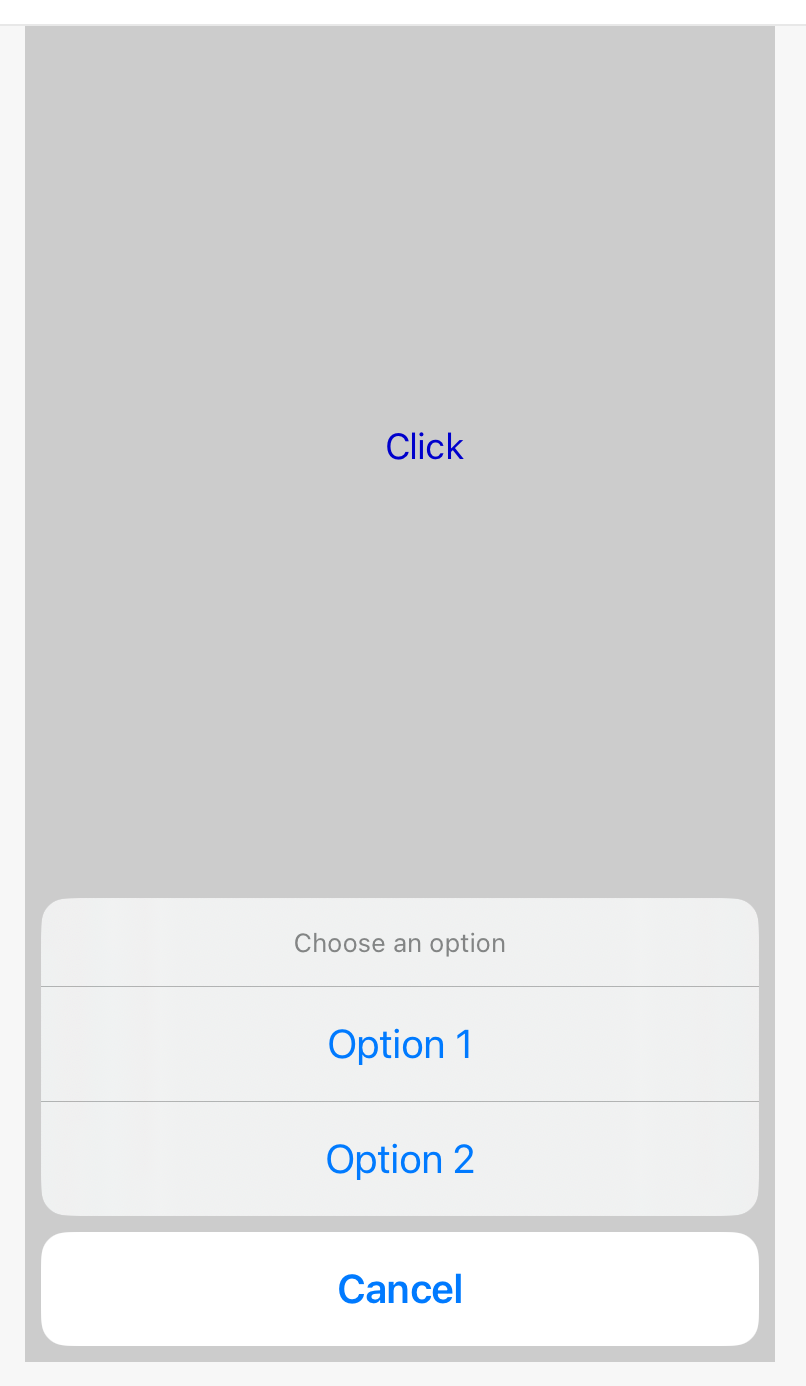
SwiftUI
To present sheet in SwiftUI, follow the below example:
import SwiftUI
import PlaygroundSupport
struct ContentView: View {
@State private var showActionSheet = false
@State private var selectedOption: String?
var body: some View {
Button("Show action sheet") {
self.showActionSheet = true
}
.actionSheet(isPresented: $showActionSheet) {
ActionSheet(
title: Text("Choose an option"),
message: Text("This is a message"),
buttons: [
.default(Text("Option 1")) {
self.selectedOption = "Option 1"
},
.default(Text("Option 2")) {
self.selectedOption = "Option 2"
},
.cancel()
]
)
}
}
}
PlaygroundPage.current.setLiveView(
ContentView()
.frame(width: 400, height: 700, alignment: .center)
)
Similarly we have:
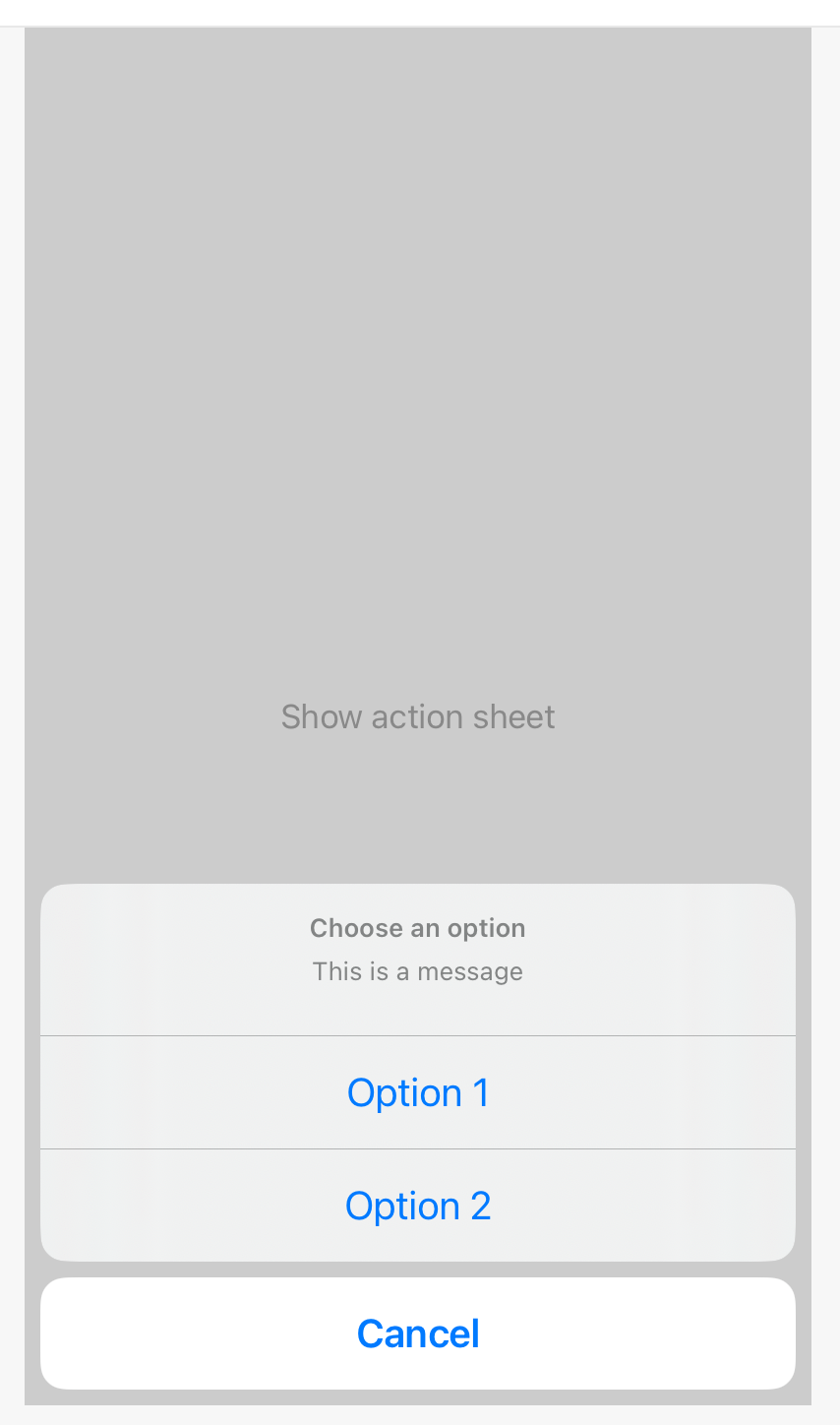