Property in Swift
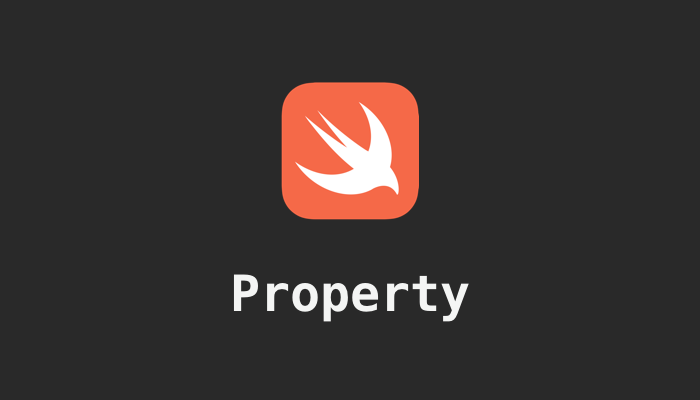
Unlike other programming languages, there are three types properties in Swift.
Stored Properties
They are constants or variables of a class, and part of the class instances that used to store values. One of the examples is the model class.
class Car {
var name: String
var price: Float = 0.0
init(name: String, price: Float) {
self.name = name
self.price = price
}
}
name
and price
are stored properties for class Car
. We can give stored properties a default value, assign values through initialization and access through .
:
let car = Car(name: "Lexus", price: 1000.0)
car.price = 2000.0
Computed Properties
class Car {
var name: String
var price: Float = 0.0
var minPrice: Float = 1000.0
var maxPrice: Float = 3000.0
var averagePrice: Float {
get {
return (minPrice+maxPrice)/2.0
}
set {
self.averagePrice = newValue
}
}
init(name: String, price: Float) {
self.name = name
self.price = price
}
}
Computed properties don't store the actual value, but provide a getter
for the access or setter
for set values. If a computed property only provides a getter
which is read only.
Static Properties
Static properties are class related not instance so we can access a static property without creating a class instance. This is useful when you want to define a util class
class CarHelper {
static var vendors: [String] = ["Lexus", "BMW", "Toyota"]
}
print(Car.vendors)