UITraitCollection
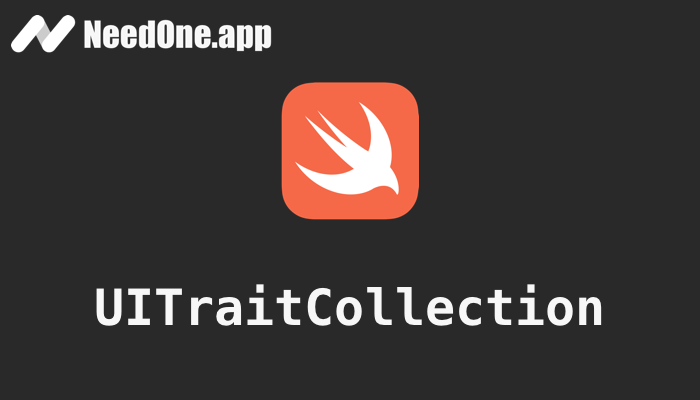
UITraitCollection is a class in the UIKit framework that defines a set of interface traits that specify the environment in which your app is running. These traits include the device’s display scale, user interface style, and size class. You can use the information contained in a UITraitCollection object to adjust the appearance and behavior of your app’s interface elements accordingly.
How to use UITraitCollection
To use UITraitCollection, you first need to get a reference to the current trait collection for your app’s interface. You can do this by calling the traitCollection
property of your view controller. This property returns an object of type UITraitCollection, which you can use to determine the current trait values for your app.
Once you have a reference to the current trait collection, you can use it to adjust the appearance and behavior of your app’s interface elements. For example, if you want to change the font size of a label based on the current display scale, you can use the displayScale
property of the trait collection to determine the appropriate font size.
Here’s an example of how you might use UITraitCollection in your app:
let traitCollection = self.traitCollection
if traitCollection.displayScale == 2.0 {
// Use a larger font size for high-resolution displays
label.font = UIFont.systemFont(ofSize: 20.0)
} else {
// Use a smaller font size for low-resolution displays
label.font = UIFont.systemFont(ofSize: 10.0)
}
Additionally, you can use the traitCollectionDidChange(_:)
method of your view controller to be notified whenever the trait collection for your app’s interface changes. This method is called automatically by the system whenever the trait collection changes, allowing you to update your app’s interface in response.
Here’s an example of how you might use the traitCollectionDidChange(_:)
method in your view controller to be notified when the trait collection changes:
override func traitCollectionDidChange(_ previousTraitCollection: UITraitCollection?) {
let traitCollection = self.traitCollection
if traitCollection.userInterfaceStyle == .dark {
// Update the interface to use a dark color scheme
self.view.backgroundColor = .black
self.label.textColor = .white
} else {
// Update the interface to use a light color scheme
self.view.backgroundColor = .white
self.label.textColor = .black
}
}
n this example, the traitCollectionDidChange(_:)
method is called whenever the user interface style of the app changes (e.g., from light to dark). When this happens, the method updates the background color and text color of the view and label accordingly. This allows the app to automatically adjust its appearance based on the current interface traits.