@AppStorage vs. @UserDefault in SwiftUI
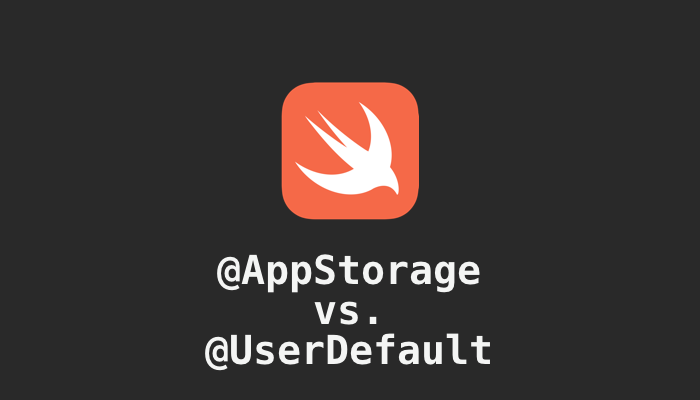
Both the @AppStorage
and @UserDefault
property wrappers in SwiftUI allow you to store and retrieve data using UserDefaults. However, there are a few key differences between the two:
@AppStorage
In SwiftUI, you can use the @AppStorage
property wrapper to store and retrieve data using UserDefaults. The @AppStorage
property wrapper binds a value to a key in UserDefaults, and updates the value in UserDefaults whenever the value changes.
Here is an example of how to use the @AppStorage
property wrapper to store and retrieve a string in UserDefaults:
struct ContentView: View {
@AppStorage("username") var username: String = ""
var body: some View {
VStack {
TextField("Enter your username", text: $username)
Text("Username: \(username)")
}
}
}
In this example, the username
property is bound to the "username" key in UserDefaults. When the user enters a new username in the text field, the value is updated in UserDefaults. The value of the username
property is also displayed in the view.
You can also use the @AppStorage
property wrapper to store and retrieve other types of data, such as integers, booleans, and arrays. Here is an example of how to store and retrieve an array, notice that if you have custom objects here, you need to have the custom struct/class conforms to Codable
.
struct ContentView: View {
@AppStorage("favoriteFruits") var favoriteFruits: [String] = []
var body: some View {
VStack {
ForEach(favoriteFruits, id: \.self) { fruit in
Text(fruit)
}
Button("Add fruit") {
self.favoriteFruits.append("apple")
}
}
}
}
In this example, the favoriteFruits
property is bound to the "favoriteFruits" key in UserDefaults. When the user taps the button, a new fruit is added to the array and the value is updated in UserDefaults. The array is displayed in the view using a ForEach
loop.
@UserDefault
The @UserDefault
property wrapper in SwiftUI allows you to store and retrieve data using UserDefaults. Like the @AppStorage
property wrapper, the @UserDefault
property wrapper binds a value to a key in UserDefaults, and updates the value in UserDefaults whenever the value changes.
Here is an example of how to use the @UserDefault
property wrapper to store and retrieve an array of strings in UserDefaults:
struct ContentView: View {
@UserDefault("favoriteFruits") var favoriteFruits: [String] = []
var body: some View {
VStack {
ForEach(favoriteFruits, id: \.self) { fruit in
Text(fruit)
}
Button("Add fruit") {
self.favoriteFruits.append("apple")
}
}
}
}
Summary
- Initial Value: The
@AppStorage
property wrapper requires you to specify an initial value for the property, which is used if the key does not exist in UserDefaults. The@UserDefault
property wrapper does not require an initial value, and will use the default value for the type if the key does not exist in UserDefaults. - Key: The
@AppStorage
property wrapper requires you to specify a key as a string literal. The@UserDefault
property wrapper allows you to specify a key using a string or aKeyPath
, which can be convenient if you want to use a computed property as the key. - Type Safety: The
@AppStorage
property wrapper does not enforce type safety, which means that you can store any type of data using any key. The@UserDefault
property wrapper enforces type safety, which means that the type of the property must match the type of the data stored in UserDefaults.
Overall, the @AppStorage
property wrapper is more flexible, but the @UserDefault
property wrapper is more type-safe and can be more convenient to use in some situations.