Introduction
Due to some requirements from work, I was asked to immigrate current iOS product into Android. If you are like me, who are mainly working on iOS, but want to put hands on Android too, this is a post can help you to understand the fundamental concept on Android.
First, the following is the workflow of Android application status, including:
Active or Running
: user tapped and run this applicationPaused
: application goes to sleep, partially hidden by other viewStoped or Backgrounded
: application completed obscured by other viewRestarted
: user get back to application
Lifecycle Methods
Similar to viewDidLoad
in iOS
, Android does provide equivalent methods to manage the running status.
1. OnCreate (similar to viewDidLoad)
This is the first method to be called when an activity is created. OnCreate is always overridden to perform any startup initializations that may be required by an Activity such as:
- Creating views
- Initializing variables
- Binding static data to list
2. OnStart (similar to viewDidAppear)
This method is always called by the system after OnCreate is finished. Activities may override this method if they need to perform any specific tasks right before an activity becomes visible such as refreshing current values of views within the activity. Android will call OnResume immediately after this method.
3. OnResume
The system calls this method when the Activity is ready to start interacting with the user. Activities should override this method to perform tasks such as:
- Ramping up frame rates (a common task in game building)
- Starting animations
- Listening for GPS updates
- Display any relevant alerts or dialogs
- Wire up external event handlers
OnResume is important because any operation that is done in OnPause should be un-done in OnResume, since it’s the only lifecycle method that is guaranteed to execute after OnPause when bringing the activity back to life.
4. OnPause
This method is called when the system is about to put the activity into the background or when the activity becomes partially obscured. Activities should override this method if they need to:
- Commit unsaved changes to persistent data
- Destroy or clean up other objects consuming resources
- Ramp down frame rates and pausing animations
- Unregister external event handlers or notification handlers (i.e. those that are tied to a service). This must be done to prevent Activity memory leaks.
- Likewise, if the Activity has displayed any dialogs or alerts, they must be cleaned up with the .Dismiss() method.
There are two possible lifecycle methods that will be called after OnPause:
OnResume will be called if the Activity is to be returned to the foreground.
OnStop will be called if the Activity is being placed in the background.
5. OnStop
This method is called when the activity is no longer visible to the user. This happens when one of the following occurs:
- A new activity is being started and is covering up this activity.
- An existing activity is being brought to the foreground.
- The activity is being destroyed.
OnStop may not always be called in low-memory situations, such as when Android is starved for resources and cannot properly background the Activity. For this reason, it is best not to rely on OnStop getting called when preparing an Activity for destruction. The next lifecycle methods that may be called after this one will be OnDestroy if the Activity is going away, or OnRestart if the Activity is coming back to interact with the user.
6. OnDestroy
This is the final method that is called on an Activity instance before it’s destroyed and completely removed from memory. In extreme situations Android may kill the application process that is hosting the Activity, which will result in OnDestroy not being invoked. Most Activities will not implement this method because most clean up and shut down has been done in the OnPause and OnStop methods. The OnDestroy method is typically overridden to clean up long running resources that might leak resources. An example of this might be background threads that were started in OnCreate.
7. OnRestart
This method is called after your activity has been stopped, prior to it being started again. A good example of this would be when the user presses the home button while on an activity in the application. When this happens OnPause and then OnStop methods are called, and the Activity is moved to the background but is not destroyed. If the user were then to restore the application by using the task manager or a similar application, Android will call the OnRestart method of the activity.
There are no general guidelines for what kind of logic should be implemented in OnRestart. This is because OnStart is always invoked regardless of whether the Activity is being created or being restarted, so any resources required by the Activity should be initialized in OnStart, rather than OnRestart.
To sum up, the above methods can be illustrated in one diagram:
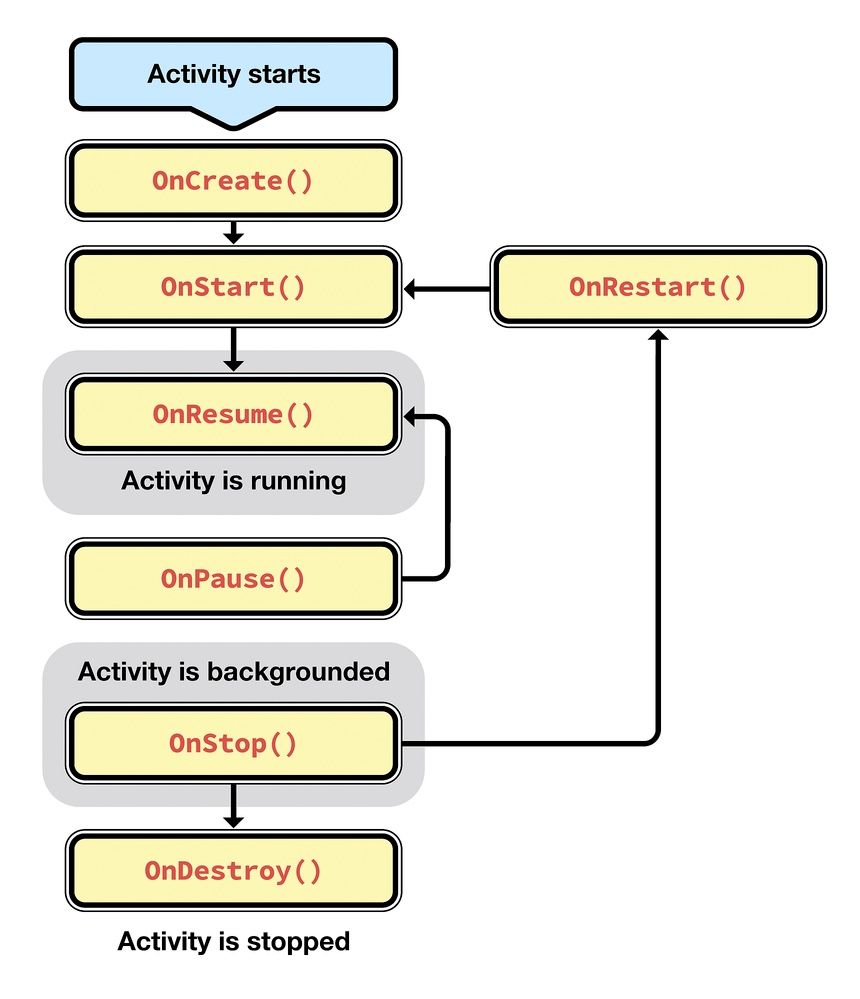
Reference
https://developer.xamarin.com/guides/android/application_fundamentals/activity_lifecycle/