Difference between activity and requireActivity() in Kotlin
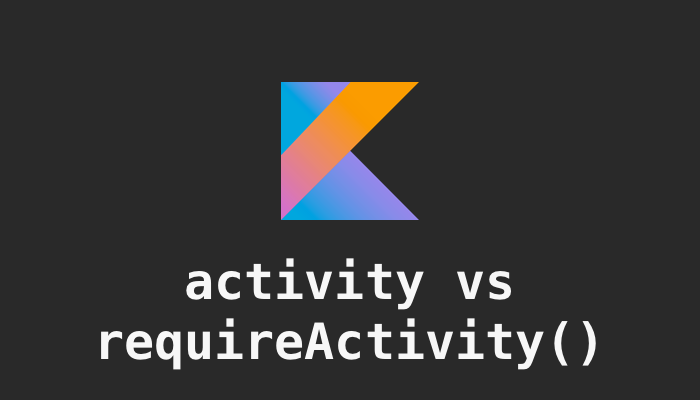
activity property
In the Android framework, the Activity
class represents an app's UI and provides an interface for the app to interact with the system. The activity
property in a Fragment
or Context
object refers to the Activity
that the Fragment
or Context
is currently associated with.
Here is an example of using the activity
property in a Fragment
to access the ActionBar
of the associated Activity
:
class MyFragment : Fragment() {
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
// Inflate the layout for this fragment
val view = inflater.inflate(R.layout.fragment_my, container, false)
// Set the title of the ActionBar
activity?.actionBar?.title = "My Fragment"
return view
}
}
In this example, the activity
property is used to access the ActionBar
of the associated Activity
. The ?
operator is used to safely access the actionBar
property in case the activity
is null.
Note: ActionBar
is deprecated in API level 28, you can use Toolbar instead.
requireActivity()
The requireActivity()
function is a convenience function that retrieves the current Activity
associated with a Fragment
or Context
, and throws an exception if the activity is null. This function is useful for ensuring that the Fragment
or Context
is associated with an Activity
before trying to interact with it.
Here is an example of using the requireActivity()
function in a Fragment
to start another Activity
:
class MyFragment : Fragment() {
override fun onViewCreated(view: View, savedInstanceState: Bundle?) {
super.onViewCreated(view, savedInstanceState)
// Find the button in the layout and set an OnClickListener
val button = view.findViewById<Button>(R.id.my_button)
button.setOnClickListener {
// Start another Activity
val intent = Intent(requireActivity(), AnotherActivity::class.java)
requireActivity().startActivity(intent)
}
}
}
In this example, the requireActivity()
function is used to retrieve the current Activity
associated with the Fragment
and start another Activity
by calling the startActivity(intent)
method on it. It will throws IllegalStateException
if there is no activity associated with this fragment. It's a safe way to use the activity instead of activity
property and doing a null check.
Note: If you want to start an activity for result, you can use startActivityForResult(intent, requestCode)
and onActivityResult(requestCode: Int, resultCode: Int, data: Intent?)
methods instead.
When should use activity or requireActivity()
The main difference between the activity
property and the requireActivity()
function in Kotlin is how they handle the case when there is no Activity
associated with a Fragment
or Context
.
- The
activity
property is a regular property that returns the currentActivity
associated with aFragment
orContext
, or null if there is no associatedActivity
. When using theactivity
property, you need to check if it's null before trying to interact with it. - The
requireActivity()
function is a convenience function that retrieves the currentActivity
associated with aFragment
orContext
, but it throws anIllegalStateException
if theactivity
is null. This function is useful for ensuring that theFragment
orContext
is associated with anActivity
before trying to interact with it.
In general, it's recommended to use requireActivity()
when you're sure that the fragment or context has an activity associated with it. This is because it will throw an exception if it is null, which will alert you to the problem, rather than silently returning null, making it harder to find the problem.