Format currency in Swift
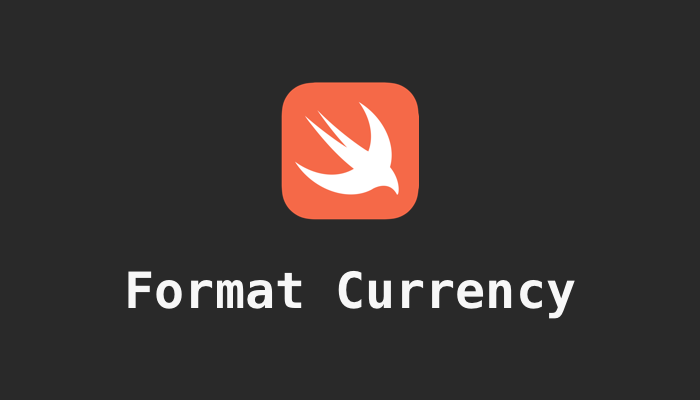
Recently I have done quite an amount of work on formatting currency in Swift project, here are some tricks on how to format number in Swift:
Format currency with auto currency symbol
By using the NumberFormatter
class, we can convert numbers into string(s), and vice versa. More conveniently, we can also configure a NumberFormatter
object to display currency values in a specific locale with a specific currency symbol automatically. For example, € in European countries, $ in Australia and son on.
let value = 100.0
let currencyFormatter = NumberFormatter()
currencyFormatter.numberStyle = .currency
currencyFormatter.locale = Locale(identifier: "en_US")
let formattedValue = currencyFormatter.string(from: value as NSNumber)
print(formattedValue) // "$100.00"
Format currency with max 2 decimals
Moreover, we can also format the currency values in specific format, such as converting 100.78 to $100.78:
let formatter = NumberFormatter()
formatter.numberStyle = .currency
formatter.minimumFractionDigits = 0
formatter.maximumFractionDigits = 2
let amount1 = 100.78
let formattedAmount1 = formatter.string(from: NSNumber(value: amount1))!
print(formattedAmount1) // Output: "$100.78"
// input is whole amount
let amount2 = 100
let formattedAmount2 = formatter.string(from: NSNumber(value: amount2))!
print(formattedAmount2) // Output: "$100"
// input is whole amount
let amount3 = 100.1
let formattedAmount3 = formatter.string(from: NSNumber(value: amount3))!
print(formattedAmount3) // Output: "$100.1"
minimumFractionDigits
meaning if the original value is whole amount or just 1 decimal part, it will only show 0 or 1 decimals, see above examples on amount2
and amount3
.
Format currency with always 2 decimals
Bear the above example in mind, we can also set up the formatter which always displaying 2 decimals (or any number of decimals you want) for currency values:
let formatter = NumberFormatter()
formatter.numberStyle = .currency
formatter.minimumFractionDigits = 2
formatter.maximumFractionDigits = 2
let amount = 123456.789
let formattedAmount = formatter.string(from: NSNumber(value: amount))!
print(formattedAmount) // Output: "$123,456.79"