Generic type vs. Any in Swift
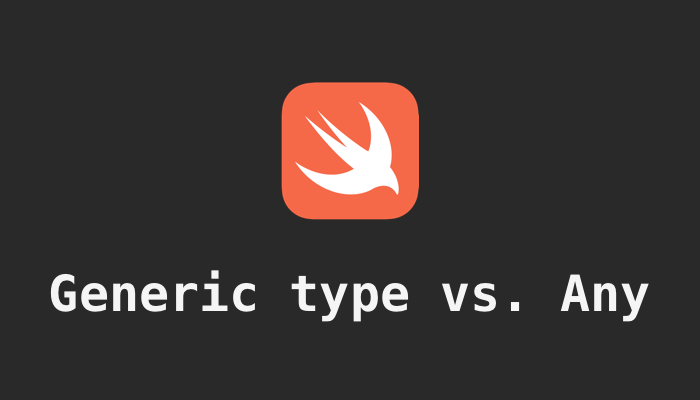
Generic type
In Swift, a generic type is a placeholder for a specific type that is specified when the generic type is used. A generic type allows you to write flexible and reusable code that can work with any type, while still taking advantage of type safety.
For example, you can use a generic type to create a function that can take any type of argument and return any type of value:
func swap<T>(a: inout T, b: inout T) {
let temp = a
a = b
b = temp
}
n this example, the swap
function takes two arguments of type T
, which is a placeholder for a specific type that will be specified when the function is called. The function can be called with any type of arguments, as long as they are the same type:
var a = 1
var b = 2
swap(&a, &b) // a = 2, b = 1
var x = "hello"
var y = "world"
swap(&x, &y) // x = "world", y = "hello"
Any
The Any
type in Swift is a type that represents any type, similar to the Object
type in other languages. The Any
type can be used to store values of any type, but it does not provide any type safety or type inference.
For example, you can use the Any
type to create an array that can store values of any type:
let array: [Any] = [1, "hello", true]
However, you will need to use type casting to access the values in the array, because the type of each value is not known at compile time:
let first = array[0] as! Int
let second = array[1] as! String
let third = array[2] as! Bool
In general, it is recommended to use generic types instead of the Any
type whenever possible, because generic types provide more type safety and type inference. However, the Any
type can be useful in certain situations where you need to store values of mixed types.
Difference between Generic type and Any in Swift
Here are some key differences between generic types and the Any
type in Swift:
- Type Safety: Generic types provide type safety, because the specific type is specified when the generic type is used. The
Any
type does not provide any type safety, because it can store values of any type. - Type Inference: Generic types provide type inference, which means that the type of the values is inferred from the context in which the generic type is used. The
Any
type does not provide any type inference, because it can store values of any type. - Type Casting: When you use a generic type, you do not need to use type casting to access the values, because the type of the values is known at compile time. When you use the
Any
type, you need to use type casting to access the values, because the type of the values is not known at compile time.